
Welcome back! In this blog, I'll demonstrate how you can leverage PowerShell to automate the entire setup of a Windows domain environment on AWS services, from creating the VPC to configuring the EC2 encrypted volumes.
Before we start, deploying this will incur AWS costs, the instance type is t3.medium and the volume is set to $ebsVolType = "io1" and $ebsIops = 1000
This is Part 1 of a 2 parter, and it will focus on setting up the scripting environment and meeting the prerequisites.
The ultimate goal is to deploy a public-facing Remote Desktop Server (RDS) and a private Domain Controller (DC) by PowerShell. The Remote Desktop Server will serve as a jump box, providing remote access to the network, while the Domain Controller will be securely tucked away in a private subnet, only accessible through the RDS.
Prerequisites
There are a few prerequisites before to deploy EC2 instances from Powershell:
PowerShell version 7 or Visual Code Studio is required
An AWS Account and its corresponding Access ID and Secret Key.
The AWS account requires the AdministratorAccess' role or delegated permissions.
A basic understanding of both AWS and Windows Domains.
The default password for the EC2 Instances is 'ChangeMe1234'.
Previous post on automating Domain and OU creation
Before diving into this blog, I highly recommend checking out the previous blogs where I used PowerShell to deploy a domain and create an Organizational Unit (OU) structure. The script used for this AWS blog is a slightly customized version of the Domain script below and as such doesn't require downloading.
The description
https://www.tenaka.net/post/deploy-domain-with-powershell-and-json-part-1
The Original Domain script
https://github.com/Tenaka/Active-Directory-Automated-Deployment-and-Delegation
Install Visual Code Studio or PowerShell
I recommend installing either PowerShell 7 (PS7) or Visual Studio Code (VSC), along with the latest .NET SDK.
.NET SDKs for Visual Studio
https://dotnet.microsoft.com/en-us/download/visual-studio-sdks
Download Visual Studio Code
https://code.visualstudio.com/download
Installing PowerShell on Windows
https://learn.microsoft.com/en-us/powershell/scripting/install/installing-powershell
AWS Account and permissions\Access ID
From within the AWS console, navigate to IAM and create a service account specifically for executing scripts to create the required AWS services.
Ensure this service account has the necessary permissions by adding the following policies and the two custom policies.
AmazonEC2FullAccess,
AmazonS3FullAccess,
AWSKeyManagementServicePowerUser,
AmazonSSMReadOnlyAccess,
AWSKeyManagementServicePowerUser,
IAMFullAccess,
AmazonSSMManagedInstanceCore
KMS Policy to grant enabling EC2 encrypted volumes, this policy requires further tweaking as it's far too encompassing.
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "VisualEditor0",
"Effect": "Allow",
"Action": [
"kms:Decrypt",
"kms:GenerateRandom",
"kms:ListRetirableGrants",
"kms:CreateCustomKeyStore",
"kms:DescribeCustomKeyStores",
"kms:ListKeys",
"kms:DeleteCustomKeyStore",
"kms:UpdateCustomKeyStore",
"kms:Encrypt",
"kms:ListAliases",
"kms:GenerateDataKey",
"kms:DisconnectCustomKeyStore",
"kms:CreateKey",
"kms:DescribeKey",
"kms:ConnectCustomKeyStore",
"kms:CreateGrant"
],
"Resource": "*"
},
{
"Sid": "VisualEditor1",
"Effect": "Allow",
"Action": "kms:*",
"Resource": "*"
}
]
}
Additionally, Session Manager rights are needed.
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"ssm:SendCommand",
"ssmmessages:CreateDataChannel",
"ssmmessages:OpenDataChannel",
"ssmmessages:OpenControlChannel",
"ssmmessages:CreateControlChannel"
],
"Resource": "*"
}
]
}
If nothing else works, consider adding the 'AdministratorAccess' policy to the service account.
Create Access Key
Create an Access Key by navigating to the Security tab of the service account and creating a 'Command Line Interface' (CLI) use case.
Record the Access Key and Secret Access Key.
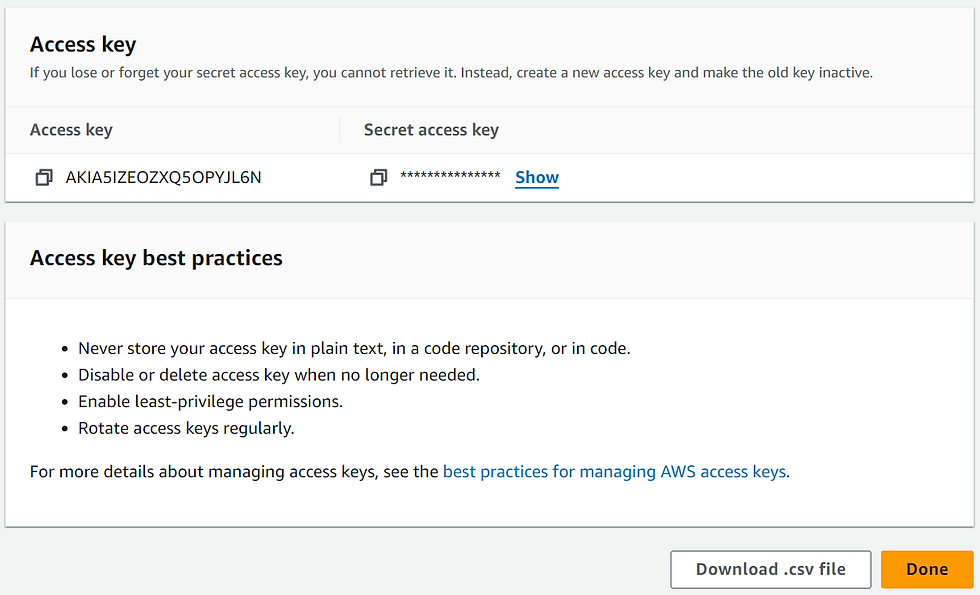
Download this script...
After you've familiarized yourself with the above concepts covered in our previous blogs and created the AWS account with the correct rights, download the PowerShell DeployVPCwithDomain.ps1 script from the link below.
This script is designed to automate the setup of EC2 instances, including a public-facing Remote Desktop Server and a secure, private domain controller.
Pick your Scripting Engine
I'll be using an elevated Visual Studio Code (VSC) session, all testing has been completed with VSC. While PowerShell version 7 should work, it hasn’t been extensively tested.
Variables that need your attention
Open the DeployVPCwithDomain.ps1 script in Visual Studio Code (VSC), but hold off on executing it. There are sections you might want to modify first.
Update the Region, the default is 'us-east-1'
$region1 = "us-east-1"
Set-defaultAWSRegion -Region $region1
Update the second and third octets of the CIDR block, as these will form the foundation for your VPC. 10.1.250.0/24 is for a future iteration where Transit Gateways are deployed for additional AD Sites. For now, 10.1.250.0/24 is free to use.
$cidr = "10.1.1" # Dont use "10.1.250.0/24"
$cidrFull = "$($cidr).0/24"
During the execution of DeployVPCwithDomain.ps1, an additional Active Directory script is downloaded from GitHub. This script is used for the configuration of the Domain Controller.
$domainZip = "https://github.com/Tenaka/AWS-PowerShell/raw/main/AD-AWS.zip"
Invoke-WebRequest -Uri $domainZip -OutFile "$($pwdPath)\AD-AWS.zip" -errorAction Stop
DeployVPCwithDomain.ps1, will pause at this point to allow updates to dcPromo.json contained within AD-AWS.zip, this is so the default password of ChangeMe1234 can be changed.
If you decide to change the default password, be sure to update it in the UserData sections for both the private and public EC2 instances as well.
Set-LocalUser -Name "administrator" -Password (ConvertTo-SecureString -AsPlainText ChangeMe1234 -Force)
That's it for now...
That's it for this blog, we're all prepped for executing the script! Make sure to come back for Part 2, where I dive into the specifics of what the script creates in AWS.
We'll also explore how the script sets up a fully functional Active Directory environment, complete with a domain controller and remote access configurations. Stay tuned!
Comments